I wrote a small Console application in C# that will nuke your logical processors (by nuke, I mean to say that it will eat your CPU utilization, and you’ll see each core spool up to 100% usage):
using System; using System.Runtime.InteropServices; using System.Threading; namespace CPUHog { class Program { [DllImport("kernel32.dll")] static extern IntPtr GetCurrentThread(); [DllImport("kernel32.dll")] static extern IntPtr SetThreadAffinityMask(IntPtr hThread, IntPtr dwThreadAffinityMask); static void Main(string[] args) { Console.WriteLine("Starting the CPU hog."); Console.WriteLine("Number of logical processors: {0}", Environment.ProcessorCount); for (int i = 0; i < Environment.ProcessorCount; i++) { SpoolThread(i); } Console.WriteLine("Press any key to exit the CPU hog."); Console.ReadLine(); Environment.Exit(0); } // Spools a spinning thread on a given CPU core. static void SpoolThread(int core) { new Thread(() => { Thread.BeginThreadAffinity(); Console.WriteLine("Nuking logical processor {0}.", core); SetThreadAffinityMask(GetCurrentThread(), new IntPtr(1 << (int)(core))); // The while loop below is what actually does the nuking. while (true) { } }).Start(); } } }
To view its effects, use Task Manager (CTRL + SHIFT + ESCAPE) -> Performance tab -> Click on CPU -> Right click the graph on the right-hand side (in the CPU view) -> Change graph to -> Logical processors. You’ll see something like this:
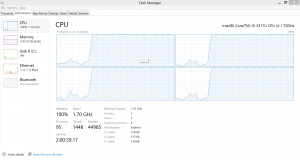